Hello Friends,
Today we will going to learn to how to create a map in android for google maps V2 .
But before that, i would like to tell you that google had deprecated their google map v1 from 3rd december 2012 onwards.It means that we unable create map api key for google map v1.(It will fully removed from 3rd march 2013) for more info: check following link
Google map V1 is deprecated :
Google Map api V2 is good thing to interact with new level of google's new map beby... :)
they provide good documentation to develop your new map app which is based on google map v2 api.
Above link contain good stuff to create your android app which is based on map v2.
I would like to share my experience, how i play with google maps v2 for android as follows
1) I create a project in ecilpse with name of MyMap.
2) then i downloded google play services by using sdk manager.I hope you are aware of it how to download google play service ???
if not,then refer image below
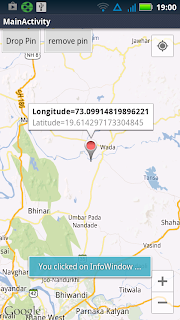
Today we will going to learn to how to create a map in android for google maps V2 .
But before that, i would like to tell you that google had deprecated their google map v1 from 3rd december 2012 onwards.It means that we unable create map api key for google map v1.(It will fully removed from 3rd march 2013) for more info: check following link
Google map V1 is deprecated :
Google Map api V2 is good thing to interact with new level of google's new map beby... :)
they provide good documentation to develop your new map app which is based on google map v2 api.
Above link contain good stuff to create your android app which is based on map v2.
I would like to share my experience, how i play with google maps v2 for android as follows
1) I create a project in ecilpse with name of MyMap.
2) then i downloded google play services by using sdk manager.I hope you are aware of it how to download google play service ???
if not,then refer image below
After Dowloading completes,These packages get stored in yours android sdk-windows/extras/google folder.we have to use these folder in our project as library so we can use google play services features in our app.
3) In ecilpse i click on file->Import->Android->Existing Android Code into workspace & I Browse my downloaded google play service folder which is in android-sdk-windows/.extras/google.
4) Or in simple way,I copied these google play services folder into my project MyMap folder's lib folder, & in property i change my compiler level 1.6 & attached library of google play service.
5) now the actual role comes here, as for obtaining mapv1 api key we used sha1 key samely we working for map v2. as follows
use cmd & find your sha1 key, for more info refer these
After that open Google APIs Console then make it on Google Maps Android V2 in services.
Click on, API Access-> Create new Android Key & create your api key using SHA-1 fingerprint & your desirable(here,my package name is com.examples.mymap) For more info check below
6) now lets move with the code,
then i use some permissions in my Android Manifest.xml such as ,below
<permission
android:name="com.examples.mymap.permission.MAPS_RECEIVE"
android:protectionLevel="signature" />
<uses-permission android:name="com.examples.mymap.permission.MAPS_RECEIVE" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="com.google.android.providers.gsf.permission.READ_GSERVICES" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-feature
android:glEsVersion="0x00020000"
android:required="true" />
& in side <application> tag & in the end of <activity> tag i used
<meta-data
android:name="com.google.android.maps.v2.API_KEY"
android:value="AIzaSyDphuv0Qi43bgLM8TkYxCoUMr4MEcNIWLo" />
7)Inside activity_main.xml i used ,
<?xml version="1.0" encoding="utf-8"?>
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/map"
android:name="com.google.android.gms.maps.SupportMapFragment"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent" >
<Button
android:id="@+id/droppin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Drop Pin" />
<Button
android:id="@+id/pinremove"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="remove pin" />
</LinearLayout>
</fragment>
8)In MainAcitivity.java i added few lines of code as below,
package com.examples.mymap;
import android.content.Context;
import android.graphics.Point;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.os.Bundle;
import android.view.Display;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.common.GooglePlayServicesUtil;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.GoogleMap.OnInfoWindowClickListener;
import com.google.android.gms.maps.GoogleMap.OnMapClickListener;
import com.google.android.gms.maps.GoogleMap.OnMarkerClickListener;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.Marker;
import com.google.android.gms.maps.model.MarkerOptions;
import com.google.android.maps.MapController;
public class MainActivity extends android.support.v4.app.FragmentActivity
implements OnMapClickListener, LocationListener, OnMarkerClickListener,
OnClickListener {
GoogleMap mmap;
LocationManager locationManager;
String provider;
Location location;
double latitude, longitude;
LatLng latLng;
Marker marker;
TextView mTapTextView;
Button Pinremove, Droppin;
MapController mvcontroller;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
provider = LocationManager.NETWORK_PROVIDER;
locationManager.requestLocationUpdates(provider, 0, 0,
MainActivity.this);
GooglePlayServicesUtil
.isGooglePlayServicesAvailable(getApplicationContext());
setContentView(R.layout.activity_main);
setUpMapIfNeeded();
Pinremove = (Button) findViewById(R.id.pinremove);
Pinremove.setOnClickListener(this);
Droppin = (Button) findViewById(R.id.droppin);
Droppin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Display display = getWindowManager().getDefaultDisplay();
int width = display.getWidth();
int height = display.getHeight();
Point point = new Point(width / 2, height / 2);
LatLng latLng = mmap.getProjection().fromScreenLocation(point);
System.out.println("++++++++++++++++" + width / 2 + "+++ "
+ height / 2);
marker = mmap.addMarker(new MarkerOptions().position(latLng)
.title("").snippet("").draggable(true));
marker.getPosition();
marker.showInfoWindow();
}
});
}
@Override
protected void onPause() {
// TODO Auto-generated method stub
super.onPause();
locationManager.removeUpdates(MainActivity.this);
}
@Override
protected void onResume() {
super.onResume();
setUpMapIfNeeded();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
private void setUpMapIfNeeded() {
// Do a null check to confirm that we have not already instantiated the
// map.
if (mmap == null) {
// Try to obtain the map from the SupportMapFragment.
mmap = ((SupportMapFragment) getSupportFragmentManager()
.findFragmentById(R.id.map)).getMap();
// Check if we were successful in obtaining the map.
if (mmap != null) {
setUpMap();
}
}
}
private void setUpMap() {
mmap.setOnMapClickListener(this);
mmap.setMyLocationEnabled(true);
mmap.getMyLocation();
// mmap.getMyLocation();
mmap.setOnMarkerClickListener(this);
mmap.setOnInfoWindowClickListener(new OnInfoWindowClickListener() {
@Override
public void onInfoWindowClick(Marker arg0) {
// TODO Auto-generated method stub
Toast.makeText(getApplicationContext(),
"You clicked on InfoWindow ...", Toast.LENGTH_LONG)
.show();
}
});
}
@Override
public void onMapClick(LatLng arg0) {
// TODO Auto-generated method stub
}
@Override
public void onLocationChanged(Location location) {
// TODO Auto-generated method stub
latitude = location.getLatitude();
longitude = location.getLongitude();
}
@Override
public void onProviderDisabled(String provider) {
// TODO Auto-generated method stub
}
@Override
public void onProviderEnabled(String provider) {
// TODO Auto-generated method stub
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// TODO Auto-generated method stub
}
@Override
public boolean onMarkerClick(Marker marker) {
// TODO Auto-generated method stub
marker.getPosition();
marker.showInfoWindow();
marker.setTitle("Longitude=" + marker.getPosition().longitude);
marker.setSnippet("Latitude=" + marker.getPosition().latitude);
return true;
}
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
// marker.remove();
mmap.clear();
}
}
9) & output will looks like,
output window-1
output window-2 output window-3
output window-4 output window-5
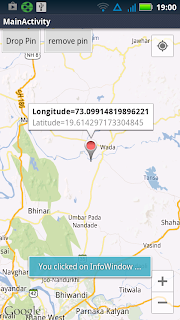
output window-6
output window-7
10) Happy Coding,Enjoy :)
Thanks you so much for this!!! Spend hours trying to get maps center coordinates. I managed to get the latitude and longitude to string like this:
ReplyDeletelatitudeTV.setText(String.valueOf(latLng));
But is there a way to get latitude and longitude in to two separate strings? Thanks very Much
Regards Jakob Harteg
Oh nevermind, I got it working my self, like this:
ReplyDeleteLatLng latLng = map.getProjection().fromScreenLocation(point);
double lat = latLng.latitude;
double lng = latLng.longitude;
String latString = String.valueOf(lat);
String lngString = String.valueOf(lng);
latitudeTV.setText("Latitude: " + latString);
longitudeTV.setText("Langitude: " + lngString);
Thanks Again
Thank You ,Harteg
Delete